I have written an ULP to auto generate the pin numbering constants for my AVR projects from the schematic net names:
https://dl.dropboxusercontent.com/u/4295670/generate_header.ulp
How to use :
1. Name all your nets in your EAGLE schematic properly like this:
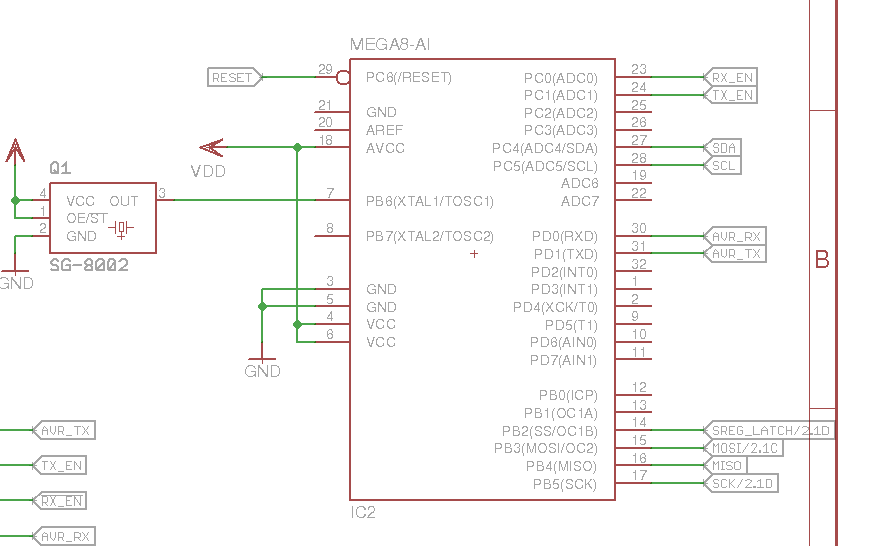
2. Run the generate_header.ulp and select the proper part.
3. Include the generated hwconfig.h file to your project.
4. You will have the follwoing structure for your each pins:
5. Use the following macros with the defines:
https://dl.dropboxusercontent.com/u/4295670/avr/global.h
https://dl.dropboxusercontent.com/u/4295670/generate_header.ulp
How to use :
1. Name all your nets in your EAGLE schematic properly like this:
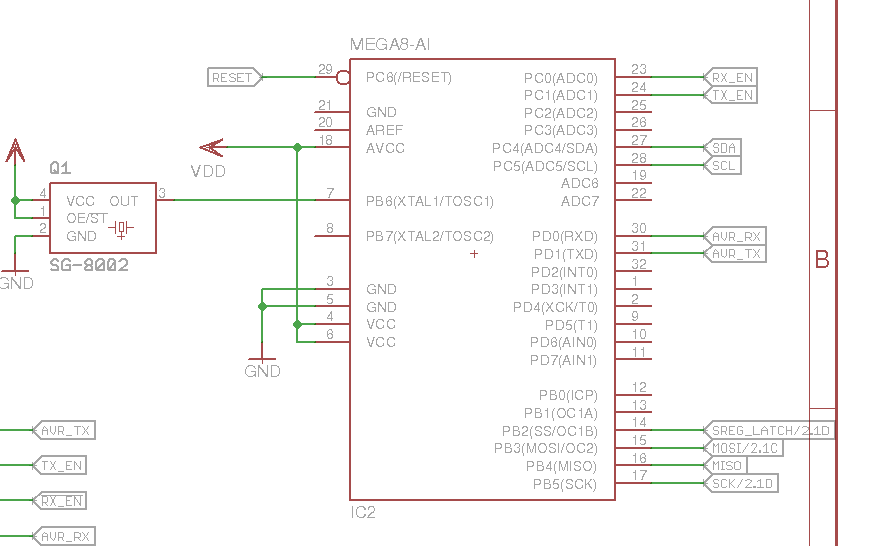
2. Run the generate_header.ulp and select the proper part.
3. Include the generated hwconfig.h file to your project.
4. You will have the follwoing structure for your each pins:
#define TX_EN_PORT PORTC
#define TX_EN_PINREG PINC
#define TX_EN_PIN 1
#define TX_EN_DDR DDRC
#define TX_EN C,1
5. Use the following macros with the defines:
// bit manipulation macros
// signals has to be defined in this way: #define LED C,1
// usage: sbi(PORT,LED1) or setting data direction: sbi(DDR,LED1)
// _BV(a) is a macro which returns the value corresponding to 2 to the power 'a'.
#define sbi_(what,x,y) what##x |= _BV(what##x##y) //set bit - using bitwise OR operator
#define cbi_(what,x,y) what##x &= ~(_BV(what##x##y)) //clear bit - using bitwise AND operator
#define tbi_(what,x,y) what##x ^= _BV(what##x##y) //toggle bit - using bitwise XOR operator
#define sbi(what,p) sbi_(what,p)
#define cbi(what,p) cbi_(what,p)
#define tbi(what,p) tbi_(what,p)
#define is_high_(x,y) ((PIN##x & _BV(PIN##x##y))) == _BV(PIN##x##y) //check if the y'th bit of register 'x' is high ... test if its AND with 1 is 1
#define is_high(p) is_high_(p)
#define is_low(p) !is_high_(p)
#define set_high_(x,y) (PORT##x |= _BV(PORT##x##y))
#define set_low_(x,y) (PORT##x &= ~_BV(PORT##x##y))
#define set_high(what) set_high_(what)
#define set_low(what) set_low_(what)
#define set_in_(x,y) DDR##x &= ~_BV(DD##x##y)
#define set_out_(x,y) DDR##x |= _BV(DD##x##y)
#define set_in(p) set_in_(p)
#define set_out(p) set_out_(p)
https://dl.dropboxusercontent.com/u/4295670/avr/global.h